Trend Catcher PineScript
//@version=5
// ---------------------------------------------------------------------
// Script Name : TrendCatcher by Robuxio
// Description : Classical Trendfollowing Strategy, please watch https://youtu.be/FJIHcBsHe_k
// Author : https://robuxio.com
// License : Free to use, modify, and distribute.
// You are welcome to adapt this code for your own purposes.
// No restrictions apply. However, please consider giving
// credit if you find it useful.
// --
strategy("Trend Catcher Strategy with Crossover", "TrendCatcher", overlay=true, precision=6, initial_capital=1000, default_qty_type=strategy.percent_of_equity, default_qty_value=100)
// ===========================
// Inputs
// ===========================
// Date Filter Inputs
start_date = input.time(timestamp("1 Jan 2019"), title="Start Date", group="Date Filter")
end_date = input.time(timestamp("1 Jan 2099"), title="End Date", group="Date Filter")
// Entry SMA Period Input
sma_entry_period = input.int(title="Entry SMA Period", defval=20, minval=1, maxval=1000, step=1, group="Entry")
// Exit SMA Period Input
sma_exit_period = input.int(title="Exit SMA Period", defval=20, minval=1, maxval=1000, step=1, group="Exit")
// BTC Filter Inputs
btc_filter = input.bool(title="Enable BTC Filter", defval=true, group="BTC Filter")
btc_sma_period = input.int(title="BTC SMA Period", defval=50, minval=1, maxval=1000, step=1, group="BTC Filter")
// Trading Direction Input
trading_direction = input.string(title="Trading Direction", defval="Both", options=["Long Only", "Short Only", "Both"], group="Trading Direction")
// ===========================
// Calculations
// ===========================
// Calculate Entry and Exit SMAs
sma_entry = ta.sma(close, sma_entry_period)
sma_exit = ta.sma(close, sma_exit_period)
// Retrieve BTC Close and SMA Values
btc_close = request.security("BINANCE:BTCUSDT", timeframe.period, close)
btc_sma_value = ta.sma(btc_close, btc_sma_period)
// Determine if BTC is Above or Below Its SMA
btc_above_sma = btc_close > btc_sma_value
btc_below_sma = btc_close < btc_sma_value
// Determine Allowed Trading Directions
is_long_allowed = (trading_direction == "Long Only") or (trading_direction == "Both")
is_short_allowed = (trading_direction == "Short Only") or (trading_direction == "Both")
// ===========================
// Entry and Exit Conditions
// ===========================
// Long Entry Condition
long_enter = is_long_allowed and (strategy.position_size == 0) and (time >= start_date) and (time < end_date) and ta.crossover(close, sma_entry) and ((btc_filter and btc_above_sma) or not btc_filter)
// Long Exit Condition
long_exit = (strategy.position_size > 0) and (close < sma_exit)
// Short Entry Condition
short_enter = is_short_allowed and (strategy.position_size == 0) and (time >= start_date) and (time < end_date) and ta.crossunder(close, sma_entry) and ((btc_filter and btc_below_sma) or not btc_filter)
// Short Exit Condition
short_exit = (strategy.position_size < 0) and (close > sma_exit)
// ===========================
// Strategy Execution
// ===========================
// Execute Long Trades
if long_exit
strategy.close("Long Entry")
if long_enter
strategy.entry("Long Entry", strategy.long, limit=close)
// Execute Short Trades
if short_exit
strategy.close("Short Entry")
if short_enter
strategy.entry("Short Entry", strategy.short, limit=close)
// ===========================
// Visual Aids
// ===========================
// Background Color Based on BTC Filter
bgcolor(btc_filter ? (btc_above_sma ? color.new(color.green, 85) : color.new(color.red, 85)) : na)
// Plot Entry and Exit SMAs
plot(sma_entry, "Entry SMA", color=color.lime)
plot(sma_exit, "Exit SMA", color=color.orange)
// Plot Shapes for Long Entries and Exits
plotshape(long_enter ? 1 : na, title="Long Entry", style=shape.triangleup, color=color.green, location=location.belowbar, size=size.normal)
plotshape(long_exit ? 1 : na, title="Long Exit", style=shape.triangledown, color=color.red, location=location.abovebar, size=size.normal)
// Plot Shapes for Short Entries and Exits
plotshape(short_enter ? 1 : na, title="Short Entry", style=shape.triangledown, color=color.red, location=location.abovebar, size=size.normal)
plotshape(short_exit ? 1 : na, title="Short Exit", style=shape.triangleup, color=color.green, location=location.belowbar, size=size.normal)
How to add to TradingView:
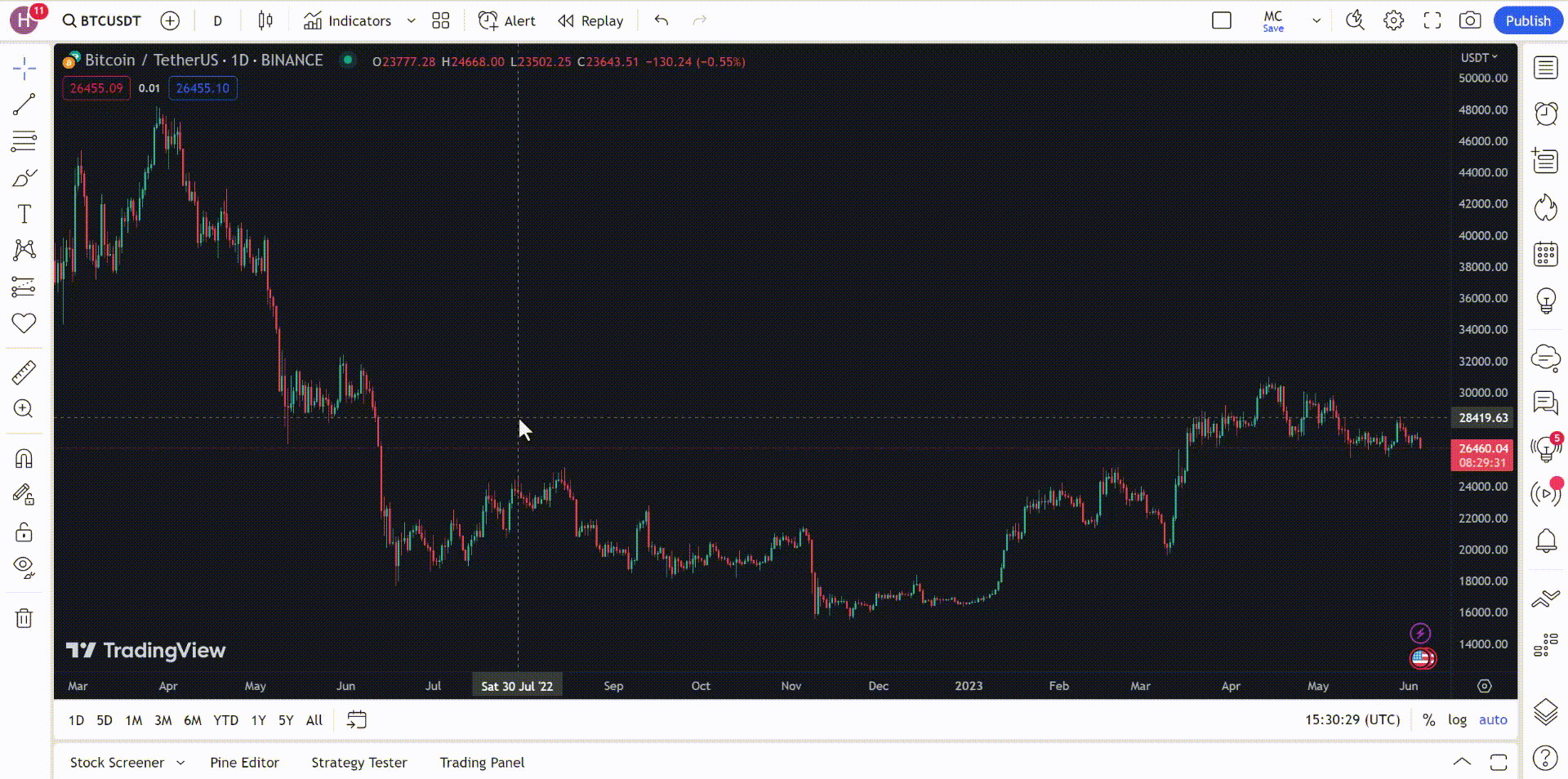
Note: While you can use Pine Script on TradingView to visualize how the strategy works, please note that this code is not suitable for live trading. TradingView requires you to handpick coins, which introduces survivorship bias—a very dangerous practice in trading.